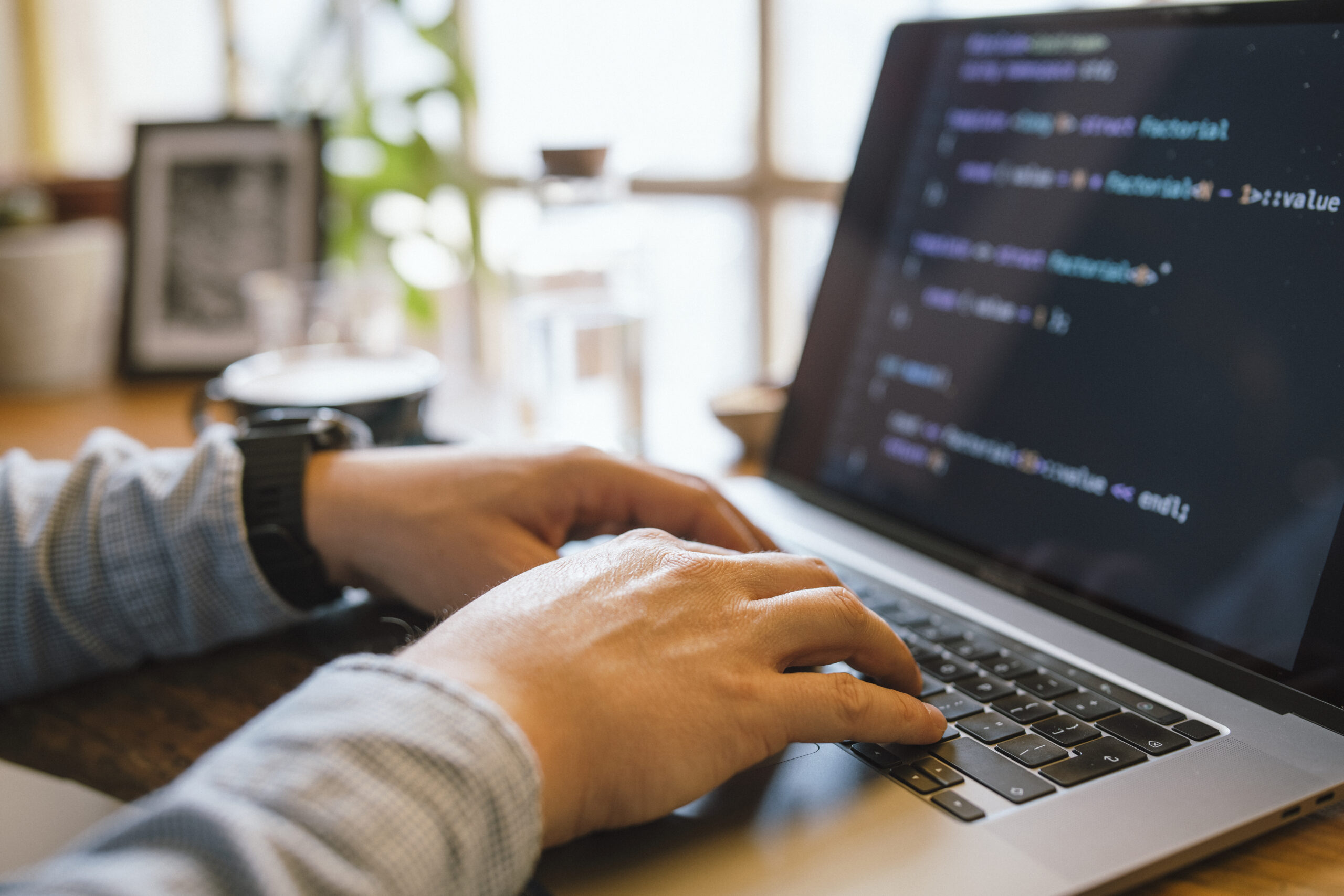
Debugging is Among the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about knowledge how and why matters go Improper, and Finding out to Consider methodically to resolve troubles successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and considerably transform your productiveness. Allow me to share many techniques to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest techniques developers can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one Element of progress, being aware of how you can connect with it proficiently for the duration of execution is equally significant. Modern-day growth environments come Geared up with strong debugging capabilities — but many builders only scratch the surface area of what these tools can perform.
Just take, by way of example, an Integrated Improvement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When applied the right way, they Enable you to observe just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into manageable jobs.
For backend or procedure-degree developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Discovering these resources could have a steeper Mastering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with version Manage programs like Git to be aware of code history, discover the exact second bugs have been launched, and isolate problematic variations.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem to make sure that when issues arise, you’re not lost in the dark. The better you realize your tools, the more time you can spend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most essential — and sometimes ignored — actions in effective debugging is reproducing the problem. Right before leaping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The difficulty? Which surroundings was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've, the easier it gets to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood environment. This might mean inputting precisely the same data, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automatic checks that replicate the edge situations or point out transitions involved. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd occur only on specific running units, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But when you finally can continually recreate the bug, you might be already halfway to fixing it. Having a reproducible scenario, You can utilize your debugging equipment far more proficiently, take a look at potential fixes safely, and communicate much more clearly together with your team or customers. It turns an abstract criticism right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what occurred, exactly where it transpired, and from time to time even why it took place — if you know how to interpret them.
Start by looking at the concept cautiously and in whole. A lot of developers, specially when beneath time pressure, look at the initial line and instantly get started creating assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and position you toward the accountable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Discovering to recognize these can substantially increase your debugging method.
Some glitches are vague or generic, and in All those cases, it’s vital to look at the context in which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These normally precede larger concerns and supply hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Prevalent logging degrees incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information and facts through progress, Details for standard activities (like effective begin-ups), Alert for probable troubles that don’t break the application, Mistake for true difficulties, and FATAL in the event the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Deal with essential occasions, point out alterations, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a perfectly-believed-out logging technique, you can decrease the time it will require to identify concerns, get further visibility into your applications, and Enhance the Over-all maintainability and reliability of one's code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, developers should technique the procedure similar to a detective resolving a mystery. This state of mind will help stop working advanced issues into manageable components and stick to clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, obtain just as much relevant information as you can with out jumping to conclusions. Use logs, test cases, and user reports to piece together a transparent photograph of what’s occurring.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications lately been produced for the codebase? Has this concern occurred before less than identical situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a managed atmosphere. If you suspect a certain operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code queries and let the final results lead you nearer to the truth.
Pay near interest to compact information. Bugs frequently disguise while in the least predicted places—just like a missing semicolon, an off-by-just one error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty with no fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Lastly, hold notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can save time for potential challenges and assist Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic issues methodically, and turn into more practical at uncovering concealed problems in intricate units.
Write Exams
Composing checks is among the best strategies to help your debugging skills and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning variations to your codebase. A nicely-tested application is easier to debug because it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific piece of logic is Doing the job as envisioned. Any time a take a look at fails, you promptly know wherever to seem, drastically lowering time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following Beforehand staying fastened.
Up coming, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance make sure a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several components or expert services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a powerful initial step. When the test fails persistently, you could give attention to correcting the bug and watch your examination go when The difficulty is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the aggravating guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Resolution immediately after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain gets to be much less effective at problem-resolving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Numerous builders report acquiring the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Master From Each and every Bug
Just about every bug you come upon is more than just A brief setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each can train you a thing valuable in the event you make time to mirror and review what went wrong.
Begin by asking oneself a number of critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The solutions generally expose blind places with your workflow or knowledge and make it easier to Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or keep a log where you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring troubles or frequent blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, aiding others steer check here clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your improvement journey. In fact, several of the best builders are not those who write best code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a new layer to the talent set. So following time you squash a bug, have a second to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — but the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep in the mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.